nodejs版本:v10.14.2 1.首先準備一個簡單的html頁面 1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>nodejs顯示html</title> 6 </head> 7 < ...
nodejs版本:v10.14.2
1.首先準備一個簡單的html頁面

1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>nodejs顯示html</title> 6 </head> 7 <body> 8 <h1>看到這句話表示html頁面成功顯示了。</h1> 9 </body> 10 </html>index.html
2.接著準備一個index.js,跟index.html放在同一目錄下
(1)導入需要用到的模塊,都是nodejs自帶的模塊
const http = require("http"), fs = require("fs"), path = require("path"), url = require("url");
創建伺服器當然需要http模塊了,fs模塊用來讀寫html的,path模塊用來獲取當前目錄的,url模塊用來解析輸入的url的
(2)獲取當前目錄
// 獲取當前目錄 var root = path.resolve();
因為index.html和index.js是放在一起的,屬於同級,直接獲取當前目錄就行了。
(3)創建伺服器
// 創建伺服器 var sever = http.createServer(function(request,response){ var pathname = url.parse(request.url).pathname; var filepath = path.join(root,pathname); // 獲取文件狀態 fs.stat(filepath,function(err,stats){ if(err){ // 發送404響應 response.writeHead(404); response.end("404 Not Found."); }else{ // 發送200響應 response.writeHead(200); // response是一個writeStream對象,fs讀取html後,可以用pipe方法直接寫入 fs.createReadStream(filepath).pipe(response); } }); }); sever.listen(8080); console.log('Sever is running at http://127.0.0.1:8080/');createServer方法創建一個sever,每次請求從request拿到url,解析後找到文件,獲取成功後寫入response。 失敗則發送404. 代碼部分到此結束,接下來是測試 (4)測試 打開cmd,找到文件所在目錄(當然用VS Code之類更方便,敲代碼測試都在一起),鍵入node index.js
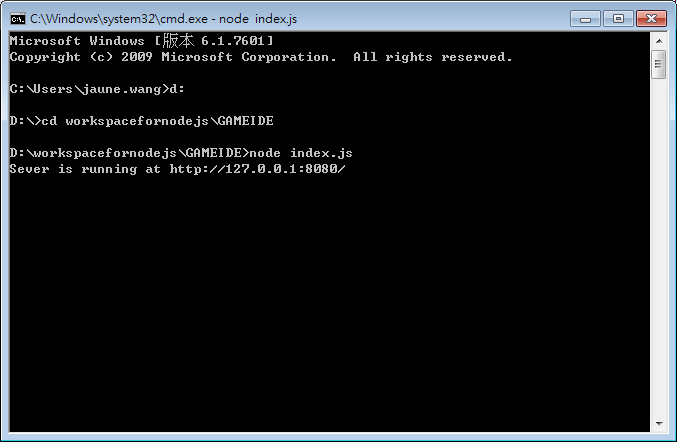
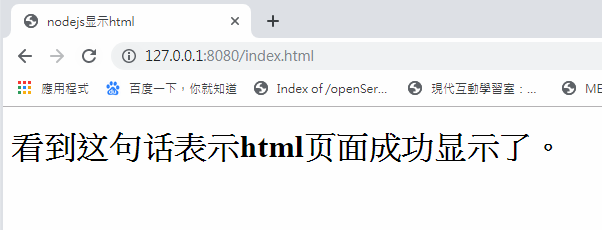

1 "use strict"; 2 const http = require("http"), 3 fs = require("fs"), 4 path = require("path"), 5 url = require("url"); 6 // 獲取當前目錄 7 var root = path.resolve(); 8 // 創建伺服器 9 var sever = http.createServer(function(request,response){ 10 var pathname = url.parse(request.url).pathname; 11 var filepath = path.join(root,pathname); 12 // 獲取文件狀態 13 fs.stat(filepath,function(err,stats){ 14 if(err){ 15 // 發送404響應 16 response.writeHead(404); 17 response.end("404 Not Found."); 18 }else{ 19 // 發送200響應 20 response.writeHead(200); 21 // response是一個writeStream對象,fs讀取html後,可以用pipe方法直接寫入 22 fs.createReadStream(filepath).pipe(response); 23 } 24 }); 25 }); 26 sever.listen(8080); 27 console.log('Sever is running at http://127.0.0.1:8080/');index.js