SpringBoot接收參數相關註解 1.基本介紹 SpringBoot接收客戶端提交數據/參數會使用到相關註解 詳解@PathVariable、@RequestHeader、@ModelAttribute、@RequestParam、@CookieValue、@RequestBody 2.接參數相 ...
SpringBoot接收參數相關註解
1.基本介紹
- SpringBoot接收客戶端提交數據/參數會使用到相關註解
- 詳解@PathVariable、@RequestHeader、@ModelAttribute、@RequestParam、@CookieValue、@RequestBody
2.接參數相關註解應用實例
演示各種方式提交數據/參數給伺服器,伺服器如何使用註解接收
2.1@PathVariable
通過@RequestMapping和@PathVariable,獲取映射路徑的占位符匹配的參數,並賦給方法形參。
index.html
<a href="/monster/100/king">@PathVariable-路徑變數 monster/100/king</a>
ParameterController.java
...
@RestController
public class ParameterController {
@GetMapping("/monster/{id}/{name}")
public String pathVariable(@PathVariable("id") Integer id,//單個接收
@PathVariable("name") String name,
//使用map可以一次接收多個路徑占位符
@PathVariable Map<String, String> map) {
System.out.println("id=" + id);
System.out.println("name=" + name);
System.out.println("map=" + map);
return "success";
}
}
訪問超鏈接,結果後臺輸出:
id=100
name=king
map={id=100, name=king}
2.2@RequestHeader
@RequestHeader用於將請求的頭信息區數據映射到功能處理方法的參數上,@RequestHeader("xxx") 代表獲取請求頭的xxx屬性,xxx不區分大小寫。
index.html
<a href="/requestHeader">@RequestHeader-獲取HTTP請求頭</a>
ParameterController.java
//@RequestHeader("xxx") 代表獲取請求頭的xxx屬性,xxx不區分大小寫
//@RequestHeader Map<String,String> header 表示將獲取請求頭的所有屬性,並放入map中
@GetMapping("/requestHeader")
public String requestHeader(@RequestHeader("Host") String host,
@RequestHeader Map<String, String> header) {
System.out.println("host=" + host);
System.out.println("header=" + header);
return "success";
}
訪問超鏈接,後臺輸出:
host=localhost:8080
header={host=localhost:8080, user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/110.0, accept=text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,*/*;q=0.8, accept-language=zh-CN,zh;q=0.8,zh-TW;q=0.7,zh-HK;q=0.5,en-US;q=0.3,en;q=0.2, accept-encoding=gzip, deflate, br, connection=keep-alive, referer=http://localhost:8080/index.html, cookie=Idea-f24e85b1=ae595c67-c988-4ef0-856d-44549b2b2eb7, upgrade-insecure-requests=1, sec-fetch-dest=document, sec-fetch-mode=navigate, sec-fetch-site=same-origin, sec-fetch-user=?1}
2.3@RequestParam
該註解的作用是:把請求中的指定名稱的參數傳遞給控制器中的形參賦值
- value / name:請求參數中的名稱 (必寫參數)
- required:請求參數中是否必須提供此參數,預設值是true,true為必須提供
- defaultValue:預設值
index.html
<a href="/hi?name=傑克&hobby=羽毛球&hobby=籃球">@RequestParam-獲取請求參數</a>
ParameterController.java
也可以使用map接收所有參數值,但是如果有多個相同名稱的參數值,只能接收第一個,因為map的key衝突了
@GetMapping("/hi")
public String hi(@RequestParam("name") String username,
@RequestParam("hobby") List<String> hobbies) {
System.out.println("username=" + username);
System.out.println("hobby=" + hobbies);
return "success";
}
訪問超鏈接,後臺輸出:
username=傑克
hobby=[羽毛球, 籃球]
2.4@CookieValue
通過@CookieValue註解可以拿到cookie的值,它的屬性有:
- value:參數名稱
- required:是否必須
- defaultValue:預設值
ParameterController.java
/**
* 1.value="xxx"表示接收名字為xx的cookie
* 如果瀏覽器帶有對應的cookie,若後面的參數類型為String,則接收到的是對應的value
* 若後面的參數類型為Cookie,則接收到的是對應的cookie
* 2.required = false 表示該值為非必須的,預設是true
*/
@GetMapping("/cookie")
public String cookie(@CookieValue(value = "cookie_key", required = false) String val,
@CookieValue(value = "username", required = false) Cookie cookie) {
System.out.println("cookie_value=" + val);
if (cookie != null) {
System.out.println("username=" + cookie.getName() + "-" + cookie.getValue());
}
return "success";
}
瀏覽器訪問方法路徑,如果沒有對應的cookie,就會輸出null,如果有則輸出對應的cookie值:
cookie_value=hello
username=username-king
2.5@RequestBody
@RequestBody接收的參數來自requestBody中,即請求體。一般用於處理非Content-Type: application/x-www-form-urlencoded
編碼格式的數據,比如:application/json
、application/xml
等類型的數據。
就application/json
類型的數據而言,使用註解@RequestBody可以將body裡面所有的json數據傳到後端,後端再進行解析。
index.html:一般來說,@RequestBody註解一般使用在post請求中,因為前端將json數據放在了請求體中。
<h1>測試@RequestBody獲取數據,獲取post請求體</h1>
<form action="/save" method="post">
姓名:<input name="name"/><br/>
年齡:<input name="age"/><br/>
<input type="submit" value="提交"/>
</form>
ParameterController.java
@PostMapping("/save")
public String postMethod(@RequestBody String content) {
System.out.println("content=" + content);
return "success";
}
瀏覽器填寫表單,提交:
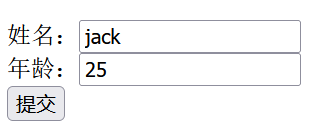
後端輸出:content=name=jack&age=25
2.6@RequestAttribute
@RequestAttribute 可以獲取request請求域中的數據
index.html
<a href="/login">@RequestAttribute-獲取request域屬性</a>
RequestController.java
package com.li.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestAttribute;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpServletRequest;
/**
* @author 李
* @version 1.0
*/
@Controller
public class RequestController {
@GetMapping("/login")
public String login(HttpServletRequest request) {
request.setAttribute("user", "Smith");//向request域中添加數據
return "forward:/ok";//轉發到/ok(需要在配置中啟用視圖解析器)
}
@GetMapping("/ok")
@ResponseBody
public String ok(@RequestAttribute(value = "user", required = false) String username) {
//獲取到request域中的數據
System.out.println("username=" + username);
return "success";
}
}
瀏覽器訪問超鏈接 /login,後臺輸出:
username=Smith
2.7@SessionAttribute
@SessionAttribute用來標註在介面的參數上,參數的值來源於session作用域。
RequestController.java
package com.li.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestAttribute;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.SessionAttribute;
import javax.servlet.http.HttpServletRequest;
/**
* @author 李
* @version 1.0
*/
@Controller
public class RequestController {
@GetMapping("/login")
public String login(HttpServletRequest request) {
//向session中添加數據
request.getSession().setAttribute("website", "https://www.baidu.com");
return "forward:/ok";//轉發到/ok(需要在配置中啟用視圖解析器)
}
@GetMapping("/ok")
@ResponseBody
public String ok(@SessionAttribute(value = "website", required = false) String site) {
//獲取session域的數據
System.out.println("site=" + site);
return "success";
}
}
瀏覽器訪問/login,後臺輸出:
site=https://www.baidu.com
3.複雜參數的使用
3.1基本介紹
- 在開發中,SpringBoot在響應客戶端請求時也支持複雜參數
- Map、Model、Errors/BindingResult、RedirectAttributes、ServletResponse、SessionStatus、UriComponentsBuilder、ServletUriComponentsBuilder、HttpSession
- Map、Model數據會被放在request域中
- RedirectAttributes重定向攜帶數據
3.2複雜參數應用實例
說明:演示覆雜參數的使用,重點演示Map、Model、ServletResponse
控制器中添加兩個方法
//模擬響應一個註冊的請求
@GetMapping("/register")
public String register(Map<String, Object> map,
Model model,
HttpServletResponse response) {
//如果有一個註冊請求,會將註冊數據封裝到map或者model中(這裡沒有寫表單,手動添加一下)
map.put("user", "jack");
map.put("job", "java後端");
model.addAttribute("salary", 10000);
//map和model中的數據會被自動放入到request域中
//演示創建cookie,通過response添加到客戶端
Cookie cookie = new Cookie("email","[email protected]");
response.addCookie(cookie);
return "forward:/registerOk";//請求轉發
}
@GetMapping("/registerOk")
@ResponseBody
public String regOk(HttpServletRequest request) {
System.out.println("user=" + request.getAttribute("user"));
System.out.println("job=" + request.getAttribute("job"));
System.out.println("salary=" + request.getAttribute("salary"));
return "success";
}
瀏覽器訪問register方法,後臺輸出:
user=jack
job=java後端
salary=10000
register()方法通過response參數將cookie返回給瀏覽器:
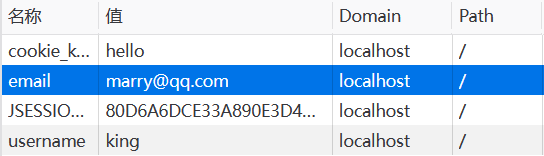
4.自定義對象參數-自動封裝
4.1基本介紹
- 在開發中,SpringBoot在響應客戶端請求時,也支持自定義對象參數
- 完成自動類型轉換與格式化
- 支持級聯封裝
4.2應用實例
演示自定義對象參數的使用,完成自動封裝,類型轉換
save.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>save</title>
</head>
<body>
<h1>根據表單數據-自動封裝POJO(級聯封裝)</h1>
<form action="/saveMonster" method="post">
編號:<input name="id" value=""/><br/>
姓名:<input name="name" value=""/><br/>
年齡:<input name="age" value=""/><br/>
婚否:<input name="isMarried" value=""/><br/>
生日:<input name="birth" value=""/><br/>
坐騎名稱:<input name="car.name" value=""/><br/>
坐騎價格:<input name="car.price" value=""/><br/>
<input type="submit" value="保存"/>
</form>
</body>
</html>
bean/Car.java
package com.li.bean;
import lombok.Data;
/**
* @author 李
* @version 1.0
*/
@Data
public class Car {
private String name;
private Double price;
}
bean/Monster.java
package com.li.bean;
import lombok.Data;
import java.util.Date;
/**
* @author 李
* @version 1.0
*/
@Data
public class Monster {
private Integer id;
private String name;
private Integer age;
private Boolean isMarried;
private Date birth;
private Car car;
}
控制器ParameterController.java添加處理請求
//處理添加Monster的方法
@PostMapping("/saveMonster")
@ResponseBody
public String saveMonster(Monster monster) {
System.out.println("monster=" + monster);
return "success";
}
瀏覽器訪問save.html,輸入數據點擊提交:
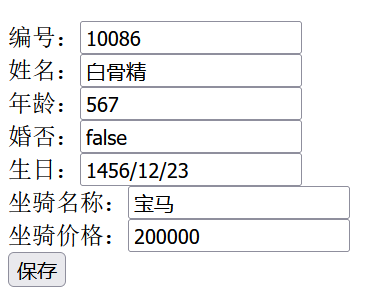
後端接收到表單信息,自動封裝到對象中(支持級聯封裝):
monster=Monster(id=10086, name=白骨精, age=567, isMarried=false, birth=Thu Dec 23 00:00:00 CST 1456, car=Car(name=寶馬, price=200000.0))