第一種方式:使用H5的API dataTransfer 實現思路: 1.為將要拖拽的元素設置允許拖拽,並賦予dragstart事件將其id轉換成數據保存; 2.為容器添加dragover屬性添加事件阻止瀏覽器預設事件,允許元素放置,並賦予drop事件進行元素的放置。 代碼如下: 第二種方式:使用原生 ...
第一種方式:使用H5的API dataTransfer
實現思路:
1.為將要拖拽的元素設置允許拖拽,並賦予dragstart事件將其id轉換成數據保存;
2.為容器添加dragover屬性添加事件阻止瀏覽器預設事件,允許元素放置,並賦予drop事件進行元素的放置。
代碼如下:
<html> <head> <meta charset="utf-8"> <style> .box1 { width: 100px; height: 100px; border: 1px black solid; margin-bottom: 20px; background: lightblue; } .box2 { width: 100px; height: 100px; border: 1px black solid; background: lightcoral; } </style> </head> <body> <!-- 參數要傳入event對象 --> <div class="box1" ondragover="allowdrop(event)" ondrop="drop(event)"> <img src="img/2.jpg" alt="00" draggable="true" ondragstart="drag(event)" id="drag" width="50" height="50"> <span>我是盒子一</span> </div> <div class="box2" ondragover="allowdrop(event)" ondrop="drop(event)"> <span>我是盒子二</span></div> <script> function allowdrop(e) { e.preventDefault(); } function drop(e) { e.preventDefault(); var data = e.dataTransfer.getData("text"); e.target.appendChild(document.getElementById(data)); } function drag(e) { e.dataTransfer.setData("text", e.target.id); } </script> </body> </html>
第二種方式:使用原生js(通過計算元素的位置結合定位實現)
思路:
1.獲取滑鼠距離元素左邊界和上邊界的距離; 2.移動後計算出元素相對原來位置的相對距離,賦予樣式。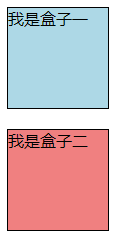
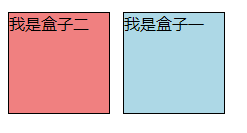
<html> <head> <meta charset="utf-8"> <style> .box1 { width: 100px; height: 100px; border: 1px black solid; margin-bottom: 20px; background: lightblue; } .box2 { width: 100px; height: 100px; border: 1px black solid; background: lightcoral; } #drag { position: relative; } </style> </head> <body> <div class="box1" id="drag"> <span>我是盒子一</span> </div> <div class="box2"> <span>我是盒子二</span></div> <script> let drag = document.querySelector("#drag");//獲取操作元素 drag.onmousedown = function (e) {//滑鼠按下觸發 var disx = e.pageX - drag.offsetLeft;//獲取滑鼠相對元素距離 var disy = e.pageY - drag.offsetTop; console.log(e.pageX); console.log(drag.offsetLeft); document.onmousemove = function (e) {//滑鼠移動觸發事件,元素移到對應為位置 drag.style.left = e.pageX - disx + 'px'; drag.style.top = e.pageY - disy + 'px'; } document.onmouseup = function(){//滑鼠抬起,清除綁定的事件,元素放置在對應的位置 document.onmousemove = null; document.onmousedown = null; }; e.preventDefault();//阻止瀏覽器的預設事件 }; </script> </body> </html>