最近學習asp.netcore 打算寫出來和大家分享,我計劃先寫Identity部分,會從開始asp.netocre identity的簡單實用開始,然後再去講解主要的類和自定義這些類。 主題:asp.netcore Identity 的簡單實用 創建項目: 使用asp.netcore 項目模版創建 ...
最近學習asp.netcore 打算寫出來和大家分享,我計劃先寫Identity部分,會從開始asp.netocre identity的簡單實用開始,然後再去講解主要的類和自定義這些類。
主題:asp.netcore Identity 的簡單實用
創建項目:
使用asp.netcore 項目模版創建一個空(empty)項目,創建完成之後編輯.csproj文件,代碼如下
<Project Sdk="Microsoft.NET.Sdk.Web"> <PropertyGroup> <TargetFramework>netcoreapp2.0</TargetFramework> </PropertyGroup> <ItemGroup> <Folder Include="wwwroot\" /> </ItemGroup> <ItemGroup> <PackageReference Include="Microsoft.AspNetCore.All" Version="2.0.0" /> <DotNetCliToolReference Include="Microsoft.EntityFrameworkCore.Tools.DotNet" Version="2.0.0" /> </ItemGroup> </Project>
增加了
<DotNetCliToolReference Include="Microsoft.EntityFrameworkCore.Tools.DotNet"
Version="2.0.0" /> 這個行代碼;
然後編輯 startup代碼文件,增加mvc,和認證部分
public void ConfigureServices(IServiceCollection services) { services.AddMvc(); } public void Configure(IApplicationBuilder app) { app.UseStatusCodePages(); app.UseDeveloperExceptionPage(); app.UseStaticFiles(); app.UseMvcWithDefaultRoute(); }
配置Identity
現在我們配置identity的信息,包括配置的修改,添加user類
1創建user類
user類代表了用戶的賬號信息,比如登陸名,密碼,角色,email等信息,代碼如下:
using Microsoft.AspNetCore.Identity; namespace DemoUser.Models { public class AppUser:IdentityUser { } }
我們是繼承了 identityuser這個類,這個類已經包含了基本的用戶信息屬性,比如 登陸名,密碼 ,手機號等,該類的反編譯之後信息如下:
// Decompiled with JetBrains decompiler // Type: Microsoft.AspNetCore.Identity.IdentityUser`1 // Assembly: Microsoft.Extensions.Identity.Stores, Version=2.0.1.0, Culture=neutral, PublicKeyToken=adb9793829ddae60 // MVID: 7E04453E-9787-4C8F-ACD1-4ADA9BB7C88C // Assembly location: /usr/local/share/dotnet/sdk/NuGetFallbackFolder/microsoft.extensions.identity.stores/2.0.1/lib/netstandard2.0/Microsoft.Extensions.Identity.Stores.dll using System; namespace Microsoft.AspNetCore.Identity { /// <summary>Represents a user in the identity system</summary> /// <typeparam name="TKey">The type used for the primary key for the user.</typeparam> public class IdentityUser<TKey> where TKey : IEquatable<TKey> { /// <summary> /// Initializes a new instance of <see cref="T:Microsoft.AspNetCore.Identity.IdentityUser`1" />. /// </summary> public IdentityUser() { } /// <summary> /// Initializes a new instance of <see cref="T:Microsoft.AspNetCore.Identity.IdentityUser`1" />. /// </summary> /// <param name="userName">The user name.</param> public IdentityUser(string userName) : this() { this.UserName = userName; } /// <summary>Gets or sets the primary key for this user.</summary> public virtual TKey Id { get; set; } /// <summary>Gets or sets the user name for this user.</summary> public virtual string UserName { get; set; } /// <summary>Gets or sets the normalized user name for this user.</summary> public virtual string NormalizedUserName { get; set; } /// <summary>Gets or sets the email address for this user.</summary> public virtual string Email { get; set; } /// <summary> /// Gets or sets the normalized email address for this user. /// </summary> public virtual string NormalizedEmail { get; set; } /// <summary> /// Gets or sets a flag indicating if a user has confirmed their email address. /// </summary> /// <value>True if the email address has been confirmed, otherwise false.</value> public virtual bool EmailConfirmed { get; set; } /// <summary> /// Gets or sets a salted and hashed representation of the password for this user. /// </summary> public virtual string PasswordHash { get; set; } /// <summary> /// A random value that must change whenever a users credentials change (password changed, login removed) /// </summary> public virtual string SecurityStamp { get; set; } /// <summary> /// A random value that must change whenever a user is persisted to the store /// </summary> public virtual string ConcurrencyStamp { get; set; } = Guid.NewGuid().ToString(); /// <summary>Gets or sets a telephone number for the user.</summary> public virtual string PhoneNumber { get; set; } /// <summary> /// Gets or sets a flag indicating if a user has confirmed their telephone address. /// </summary> /// <value>True if the telephone number has been confirmed, otherwise false.</value> public virtual bool PhoneNumberConfirmed { get; set; } /// <summary> /// Gets or sets a flag indicating if two factor authentication is enabled for this user. /// </summary> /// <value>True if 2fa is enabled, otherwise false.</value> public virtual bool TwoFactorEnabled { get; set; } /// <summary> /// Gets or sets the date and time, in UTC, when any user lockout ends. /// </summary> /// <remarks>A value in the past means the user is not locked out.</remarks> public virtual DateTimeOffset? LockoutEnd { get; set; } /// <summary> /// Gets or sets a flag indicating if the user could be locked out. /// </summary> /// <value>True if the user could be locked out, otherwise false.</value> public virtual bool LockoutEnabled { get; set; } /// <summary> /// Gets or sets the number of failed login attempts for the current user. /// </summary> public virtual int AccessFailedCount { get; set; } /// <summary>Returns the username for this user.</summary> public override string ToString() { return this.UserName; } } }
這個類已經定義好了一些關於賬戶的屬性,,假如我們覺得還需要添加自定義的屬性比如 用戶的地址,我們只需要在我們繼承的類添加自定義的屬性即可;
創建資料庫上下文:
下麵我們要創建一個數據上下文用於連接資料庫,代碼如下:
using Microsoft.AspNetCore.Identity.EntityFrameworkCore; using Microsoft.EntityFrameworkCore; namespace DemoUser.Models { public class AppIdentityDbContext :IdentityDbContext<AppUser> { public AppIdentityDbContext(DbContextOptions<AppIdentityDbContext> options) : base(options) { } } }
配置資料庫連接字元串:
為了連接資料庫我們要配置一個連接字元串,修改appsettings.json文件如下:
{ "Data": { "AppStoreIdentity": { "ConnectionString": "Server=192.168.0.4;Database=IdentityUsers;User ID =SA; Password=!@#" } } }
下麵我們需要在startup類配置連接字元串到資料庫上下文;
public void ConfigureServices(IServiceCollection services) { services.AddDbContext<AppIdentityDbContext>(options => options.UseSqlServer( Configuration["Data:AppStoreIdentity:ConnectionString"])); services.AddIdentity<AppUser, IdentityRole>() .AddEntityFrameworkStores<AppIdentityDbContext>() .AddDefaultTokenProviders(); services.AddMvc(); }
創建資料庫:
下麵我們需要用EFCore去生成一個資料庫:
打開控制台,定位到當前的項目,輸入:
dotnet ef migrations add Initial
然後就會產生一個migrations文件夾,裡面是我們要生成資料庫的代碼;
然後 在控制台輸入:
dotnet ef database update
這樣我們的資料庫就生成了
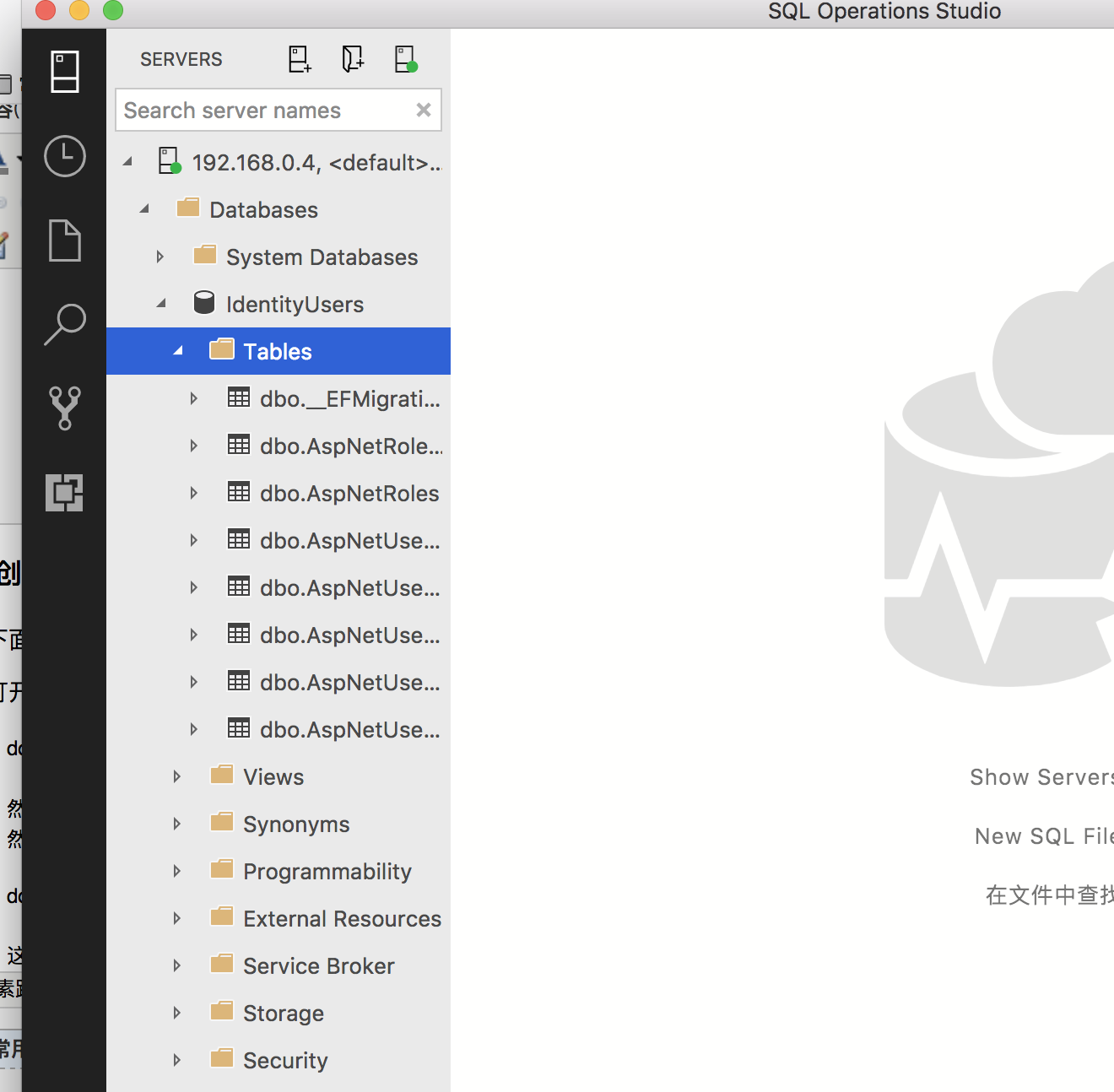
這裡是我上傳的github倉庫地址:https://github.com/bluetianx/AspnetCoreExample
後續:
我會創建一個具有增刪改查的賬戶管理界面