知識總覽 2.3.1 單鏈表的定義 知識總覽 單鏈表定義 #include<stdio.h> #include<string.h> #include<stdlib.h> struct LNode{ int data; struct LNode *next; }; int main(){ struct ...
知識總覽
2.3.1 單鏈表的定義
知識總覽
單鏈表定義
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
struct LNode{
int data;
struct LNode *next;
};
int main(){
struct LNode *p=(struct LNode*)malloc(sizeof(struct LNode));
return 0;
}
typedef重命名
typedef struct LNode{
int data;
struct LNode *next;
}LNode,*LinkList;
等同於
struct LNode{
int data;
struct LNode *next;
};
typedef struct LNode LNode;
typedef struct LNode *LinkList;
頭插法建立單鏈表
頭插法
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
typedef struct LNode{
int data;
struct LNode *next;
}LNode,*LinkList;
LinkList List_HeadInsert(LinkList &L){
LNode *s;
int x;
L=(LinkList)malloc(sizeof(LNode)); //創建頭節點
L->next=NULL; //初始為空鏈表
scanf("%d",&x); //輸入節點的值
while(x!=9999){ //輸出9999表示結束
s=(LNode*)malloc(sizeof(LNode)); //創建新節點
s->data=x;
s->next=L->next;
L->next=s; //將新節點插入表中,L為頭指針
scanf("%d",&x);
}
return L;
}
int main(){
LinkList L;
List_HeadInsert(L);
}
強調這是一個單鏈表 ————使用LinkList
強調這是一個節點 ————使用LNode*
不帶頭節點的單鏈表
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
typedef struct LNode{
int data;
struct LNode *next;
}LNode,*LinkList;
//初始化一個空的單鏈表
bool InitList(LinkList &L){
L=NULL; //空表,//防止臟數據
return true;
}
//判斷單鏈表是否為空
bool Empty(LinkList L){
return (L==NULL);
}
int main(){
LinkList L; //聲明一個指向單鏈表的指針 //此處並沒有創建一個節點
InitList(L);
Empty(L);
}
帶頭結點的單鏈表
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
typedef struct LNode{
int data;
struct LNode *next;
}LNode,*LinkList;
//初始化一個單鏈表 (帶頭結點)
bool InitList(LinkList &L){
L=(LNode*)malloc(sizeof(LNode));
if(L==NULL)
return false;
L->next=NULL;
return true;
}
//判斷單鏈表是否為空 (帶頭結點)
bool Empty(LinkList L){
if(L->next==NULL)
return true;
else
return false;
}
int main(){
LinkList L; //聲明一個指向單鏈表的指針
InitList(L);
Empty(L);
}
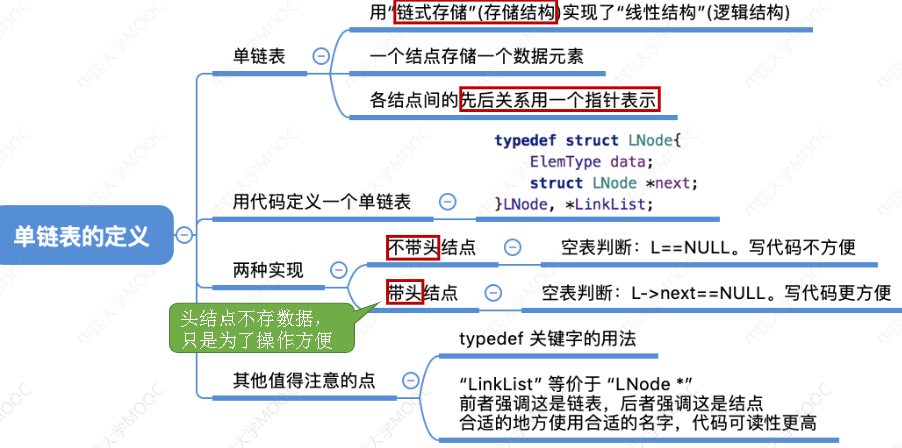
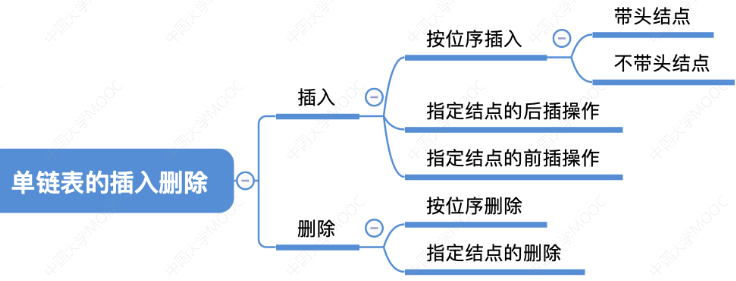
按位序插入(帶頭結點)
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
typedef struct LNode{
int data;
struct LNode *next;
}LNode,*LinkList;
//初始化一個單鏈表 (帶頭結點)
bool InitList(LinkList &L){
L=(LNode*)malloc(sizeof(LNode));
if(L==NULL)
return false;
L->next=NULL;
return true;
}
//判斷單鏈表是否為空 (帶頭結點)
bool Empty(LinkList L){
if(L->next==NULL)
return true;
else
return false;
}
//在第i個位置插入元素e(帶頭結點)
bool ListInsert(LinkList &L,int i,int e){
if(i<1)
return false;
LNode *p; //指針p指向當前掃描到的結點
int j=0; //當前p指向的是第幾個結點
p=L; //L指向頭結點,頭結點是第0個結點(不存數據)
while(p!=NULL&&j<i-1){ //迴圈找到第i-1 個結點 //找位置
p=p->next;
j++;
}
if(p==NULL) //i值不合法
return false;
LNode *s=(LNode*)malloc(sizeof(LNode));
s->data=e;
s->next=p->next;
p->next=s; //將結點s連到p之後
return true; //插入成功
}
int main(){
LinkList L; //聲明一個指向單鏈表的指針
InitList(L);
Empty(L);
ListInsert(L,1,9);
}
不帶頭節點
LNode * GetElem(LinkList L ,int i){
int j=1;
LNode *p=L->next;
if(i==0)
return L;
if(i<1)
return NULL;
while(p!=NULL&&j<i){
p=p->next;
j++;
}
本文來自博客園,作者:abandon11,轉載請註明原文鏈接:https://www.cnblogs.com/zhangsai/p/17777887.html