# Unity 如何獲取Texture 的記憶體大小 在Unity中,要獲取Texture的記憶體文件大小,可以使用UnityEditor.TextureUtil類中的一些函數。這些函數提供了獲取存儲記憶體大小和運行時記憶體大小的方法。由於UnityEditor.TextureUtil是一個內部類,我們需要 ...
Unity 如何獲取Texture 的記憶體大小
在Unity中,要獲取Texture的記憶體文件大小,可以使用UnityEditor.TextureUtil類中的一些函數。這些函數提供了獲取存儲記憶體大小和運行時記憶體大小的方法。由於UnityEditor.TextureUtil是一個內部類,我們需要使用反射來訪問它。
步驟
- 導入UnityEditor命名空間和System.Reflection命名空間:
using UnityEditor;
using System.Reflection;
- 創建一個函數來獲取Texture的記憶體文件大小:
public static long GetTextureFileSize(Texture2D texture)
{
long fileSize = 0;
// 使用反射獲取UnityEditor.TextureUtil類的Type
Type textureUtilType = typeof(TextureImporter).Assembly.GetType("UnityEditor.TextureUtil");
// 使用反射獲取UnityEditor.TextureUtil類的GetStorageMemorySizeLong方法
MethodInfo getStorageMemorySizeLongMethod = textureUtilType.GetMethod("GetStorageMemorySizeLong", BindingFlags.Static | BindingFlags.Public);
// 調用GetStorageMemorySizeLong方法獲取存儲記憶體大小
fileSize = (long)getStorageMemorySizeLongMethod.Invoke(null, new object[] { texture });
return fileSize;
}
- 創建一個函數來獲取Texture的運行時記憶體大小:
public static long GetTextureRuntimeMemorySize(Texture2D texture)
{
long memorySize = 0;
// 使用反射獲取UnityEditor.TextureUtil類的Type
Type textureUtilType = typeof(TextureImporter).Assembly.GetType("UnityEditor.TextureUtil");
// 使用反射獲取UnityEditor.TextureUtil類的GetRuntimeMemorySizeLong方法
MethodInfo getRuntimeMemorySizeLongMethod = textureUtilType.GetMethod("GetRuntimeMemorySizeLong", BindingFlags.Static | BindingFlags.Public);
// 調用GetRuntimeMemorySizeLong方法獲取運行時記憶體大小
memorySize = (long)getRuntimeMemorySizeLongMethod.Invoke(null, new object[] { texture });
return memorySize;
}
示例代碼
示例 1:獲取Texture的存儲記憶體大小
using UnityEngine;
using UnityEditor;
using System.Reflection;
public class TextureSizeExample : MonoBehaviour
{
[SerializeField]
private Texture2D texture;
private void Start()
{
long fileSize = GetTextureFileSize(texture);
Debug.Log("Texture File Size: " + fileSize + " bytes");
}
private static long GetTextureFileSize(Texture2D texture)
{
long fileSize = 0;
Type textureUtilType = typeof(TextureImporter).Assembly.GetType("UnityEditor.TextureUtil");
MethodInfo getStorageMemorySizeLongMethod = textureUtilType.GetMethod("GetStorageMemorySizeLong", BindingFlags.Static | BindingFlags.Public);
fileSize = (long)getStorageMemorySizeLongMethod.Invoke(null, new object[] { texture });
return fileSize;
}
}
示例 2:獲取Texture的運行時記憶體大小
using UnityEngine;
using UnityEditor;
using System.Reflection;
public class TextureSizeExample : MonoBehaviour
{
[SerializeField]
private Texture2D texture;
private void Start()
{
long memorySize = GetTextureRuntimeMemorySize(texture);
Debug.Log("Texture Runtime Memory Size: " + memorySize + " bytes");
}
private static long GetTextureRuntimeMemorySize(Texture2D texture)
{
long memorySize = 0;
Type textureUtilType = typeof(TextureImporter).Assembly.GetType("UnityEditor.TextureUtil");
MethodInfo getRuntimeMemorySizeLongMethod = textureUtilType.GetMethod("GetRuntimeMemorySizeLong", BindingFlags.Static | BindingFlags.Public);
memorySize = (long)getRuntimeMemorySizeLongMethod.Invoke(null, new object[] { texture });
return memorySize;
}
}
示例 3:同時獲取Texture的存儲記憶體大小和運行時記憶體大小
using UnityEngine;
using UnityEditor;
using System.Reflection;
public class TextureSizeExample : MonoBehaviour
{
[SerializeField]
private Texture2D texture;
private void Start()
{
long fileSize = GetTextureFileSize(texture);
long memorySize = GetTextureRuntimeMemorySize(texture);
Debug.Log("Texture File Size: " + fileSize + " bytes");
Debug.Log("Texture Runtime Memory Size: " + memorySize + " bytes");
}
private static long GetTextureFileSize(Texture2D texture)
{
long fileSize = 0;
Type textureUtilType = typeof(TextureImporter).Assembly.GetType("UnityEditor.TextureUtil");
MethodInfo getStorageMemorySizeLongMethod = textureUtilType.GetMethod("GetStorageMemorySizeLong", BindingFlags.Static | BindingFlags.Public);
fileSize = (long)getStorageMemorySizeLongMethod.Invoke(null, new object[] { texture });
return fileSize;
}
private static long GetTextureRuntimeMemorySize(Texture2D texture)
{
long memorySize = 0;
Type textureUtilType = typeof(TextureImporter).Assembly.GetType("UnityEditor.TextureUtil");
MethodInfo getRuntimeMemorySizeLongMethod = textureUtilType.GetMethod("GetRuntimeMemorySizeLong", BindingFlags.Static | BindingFlags.Public);
memorySize = (long)getRuntimeMemorySizeLongMethod.Invoke(null, new object[] { texture });
return memorySize;
}
}
註意事項
- 確保在使用反射訪問UnityEditor.TextureUtil類之前,已經導入了UnityEditor命名空間和System.Reflection命名空間。
- 使用反射時,需要使用BindingFlags.Static | BindingFlags.Public來獲取靜態公共方法。
- 在示例代碼中,我們使用了Texture2D類型的變數來表示Texture,你可以根據實際情況修改代碼以適應不同的Texture類型。
__EOF__
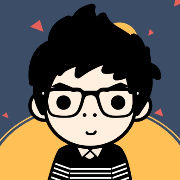
本文鏈接:
版權聲明:本博客所有文章除特別聲明外,均採用 BY-NC-SA 許可協議。轉載請註明出處!
聲援博主:如果您覺得文章對您有幫助,可以點擊文章右下角 【 推薦】 一下。您的鼓勵是博主的最大動力!