教程簡介 什麼是SEO和SEO文案 - 一個關於搜索引擎優化(SEO)入門教程,以瞭解什麼是SEO和各種SEO工具和技術,包括白帽黑帽Spamdexing和Meta標簽關鍵詞主題標題超鏈接圖像網頁優化和搜索引擎抓取索引處理相關性計算結果檢索隱藏元標記填充門口網關頁面劫持 搜索引擎優化,又稱為SEO, ...
目錄
Python類
Python類的設計原則
- 封裝(Encapsulation):Python類被設計用來將相關數據和行為封裝到一個獨立的單元中。
- 繼承(Inheritance):Python支持繼承,允許子類從父類繼承屬性和方法。有利於代碼的復用和創建相關類的層次結構。
- 多態(Polymorphism):Python支持多態,也就意味著不同類的對象可以被視為同一類型,這使得代碼的設計具有更大的靈活性。
- 組合(Composition):Python鼓勵使用組合而不是繼承,這意味著對象是由其他對象組成的,而不是由父類派生的。這可以使您的代碼更加模塊化,更易於維護。
- Duck類型(Duck typing):Python使用Duck類型,這意味著對象的類型由其行為而非類決定,這樣更容易編寫可用於各種對象的通用代碼。
- 特殊方法(Special methods):Python提供了許多特殊方法,允許為內置操作自定義行為。例如
__len__
方法允許你定義len()
函數如何處理類的對象。 - 可讀性(Readability):與Python的一般設計原則一樣,類也應該優先考慮可讀性和簡單性,也就是說,對方法和屬性使用清晰簡潔的名稱,避免不必要的複雜性。
特殊方法【Special methods】
Python中的特殊方法是一系列預定義的方法,允許你為對象上的內置操作定義自定義行為,使用雙下劃線首碼和尾碼來識別,也成為"dunder"方法。這裡講述一些Python類中常用的特殊方法:
__init__(self, ...)
: 這是構造方法,對象創建時調用,用於初始化對象的屬性並設置其初始狀態。__str__(self)
: 返回對象的字元串表示,用於創建一個人類可讀的字元串表示。__repr__(self)
: 返回對象的字元串表示,用於創建可用於創建對象的字元串表示。__len__(self)
: 返回對象的長度,供len()
函數使用。__getitem__(self, key)
: 這個方法允許你以括弧的形式訪問對象的元素,可以使用[]
來訪問元素。__setitem__(self, key, value)
: 這個方法允許你以括弧的形式設置對象元素的值,可以使用[]
來修改元素的值。__delitem__(self, key)
: 這個方法允許你以括弧的形式刪除對象的元素。__add__(self, other)
: 自定義對象的+
操作方式。__eq__(self, other)
: 自定義對象的==
操作方式。__lt__(self, other)
: 自定義對象的<
操作方式。
這隻是較為常用的一些,Python中還提供了很多其他的特殊方法。在你的自定義類中,通過定義這些方法,你可以自定義你對象的行為以更直觀、更方便地使用它們。
示例一:
class Person:
def __init__(self, name, age, items):
self.name = name
self.age = age
self.items = items
def __str__(self):
return f"{self.name} ({self.age})"
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
person = Person("Alice", 25)
print(person) # output: Alice (25)
print(repr(person)) # output: Person(name='Alice', age=25)
示例二:
class MyObject:
def __init__(self, items):
self.items = items
def __len__(self):
return len(self.items)
def __getitem__(self, index):
return self.items[index]
def __setitem__(self, index, value):
self.items[index] = value
def __delitem__(self, index):
del self.items[index]
def __add__(self, other):
new_items = self.items + other.items
return MyObject(new_items)
def __eq__(self, other):
if isinstance(other, MyObject):
return self.items == other.items
return False
def __lt__(self, other):
if isinstance(other, MyObject):
return len(self.items) < len(other.items)
return NotImplemented
obj = MyObject([1, 2, 3])
print(len(obj)) # output: 3
print(obj[1]) # output: 2
obj[1] = 4
print(obj.items) # output: [1, 4, 3]
del obj[1]
print(obj.items) # output: [1, 3]
obj1 = MyObject([1, 2])
obj2 = MyObject([3, 4])
obj3 = obj1 + obj2
print(obj3.items) # output: [1, 2, 3, 4]
obj4 = MyObject([1, 2, 3])
obj5 = MyObject([4, 5])
obj6 = MyObject([1, 2, 3])
print(obj1 == obj2) # output: False
print(obj1 == obj3) # output: True
print(obj1 < obj2) # output: False
print(obj2 < obj1) # output: True
- 格式化字元串
詳細解釋下這句話,字元串文字前面的f
用於創建格式化字元串(f-string, formatted string
),格式化字元串是一種允許你使用大括弧{}
將表達式嵌入占位符中的字元串,這些表達式將在運行時被求值並替換為它們的值。
例如下麵的代碼中,嵌入的表達式有兩個{self.name}
和{self.age}
,運行時,會將這兩個表達式替換為其值並返回。
f"Person(name='{self.name}', age={self.age})"
Duck typing
The term "duck typing" comes from the saying, "If it looks like a duck, swims like a duck, and quacks like a duck, then it probably is a duck."
Duck類型是Python等動態類型編程語言中使用的一種編程概念。在Duck類型中,一個對象的類型是由它的行為決定的,而不是它的class或者type決定的。Duck Typing一詞來源於這樣一句話“如果它看起來像鴨子,游泳像鴨子,嘎嘎叫像鴨子,那麼它可能就是鴨子”。
Duck類型使得程式員可以編寫適用於任何具有必要屬性和方法的對象的代碼,比如:
def print_size(obj):
print(len(obj))
該函數接收一個參數obj
並列印其size。由於Python使用duck類型,這個函數適用於任何包含len()
方法的對象,比如字元串、列表、元組、字典等等(string, list, tuple, dictionaries and so on)。
使用Duck類型可以編寫更加通用的代碼,使得代碼更加容易復用和維護。
但是使用Duck類型也極易出錯,為了避免這些錯誤,可是使用類型檢查來確保你的代碼運行在你期望的對象類型上。
內置函數
- 關於
len(obj)
中的len()
len()
是Python內置函數,用於返回對象的長度。
len()
可被用於多種類型的對象,包括字元串、列表、元組、字典、集合等,並返回對象中元素的數目。
在自定義的類中,通過實現特殊方法__len__()
來使用len()
函數來檢索對象的長度,返回值要求是整數。
-
關於內置函數的更多細節
上面講述的是其中一個內置函數,詳細看下,Python中的一些其他內置函數:
print()
: 列印特定信息到控制台,對應__str__()
type()
: 返回對象類型,對應__class__()
len()
: 返回對象長度,對應__len__()
range()
: 生成數字序列,預設從0開始,步長為1,直到某個終止值[不包括]input()
: 接收控制台輸入int(), float(), str()
: 將輸入轉換為某種特定類型,整數、浮點數或字元串。max(), min()
: 在一個序列中找到最大值和最小值,適用於列表、元組、集合等,對應__gt__
,__lt__()
sum()
: 取得序列值得和,適用於列表、元組、集合等sorted()
以升序排序一個序列,適用於列表、元組、集合等zip()
: 將兩個或多個序列合併為一個元組序列
示例一:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"Person(name='{self.name}', age={self.age})"
def __lt__(self, other):
return self.age < other.age
def __gt__(self, other):
return self.age > other.age
p1 = Person("Alice", 25)
p2 = Person("Bob", 30)
p3 = Person("Charlie", 20)
people = [p1, p2, p3]
print(p1) # Output: Person(name='Alice', age=25)
print(min(people).name) # Output: 'Charlie'
print(max(people).name) # Output: 'Bob'
特殊方法與內置函數的對應:
特殊方法 | 內置函數 | 備註 |
---|---|---|
__len__() |
len() |
返回對象的長度 |
__getitem__(), __setitem__() |
[] |
定義對象元素的訪問和修改行為 |
__iter__(), __next__() |
for 等 |
定義對象的迭代行為 |
__contains__() |
in |
判斷對象是否包含某個特定元素 |
__add__(), __radd__() |
+ |
定義對象的加法行為 |
__sub__(), __rsub__() |
- |
定義對象的減法行為 |
__mul__(), __rmul__() |
* |
定義對象的乘法行為 |
__divmod__(), __rdivmod__() |
divmod() |
定義對象的餘數除法行為 |
__eq__(), __ne__() |
==, != |
定義對象的判等操作 |
__lt__(), __le__(), __gt__(), __ge__() |
<, <=, >, >= |
定義對象間的比較操作 |
__hash__() |
hash() |
定義對象的哈希值(hash value) |
English Version
The key design principles for Python classes
- Encapsulation:Python classes are designed to encapsulated related data and bahavior into a single unit.
- Inheritance:Python supports inheritance, which allows classes to inherit attributes and methods from parent classes. This makes it easier to reuse code and create hierarchies of related classes.
- Polymorphism:Python supports polymorphism, which means that objects of different classes can be treated as if they are the same type. This allows for greater flexibility in the design of your code.
- Composition:Python encourages the use of composition over inheritance, which means that objects are made up of other objects rather than being derived from parent classes. This can make your code more modular and easier to maintain.
- Duck typing: Python uses duck typing, which means that the type of an object is determined by its behavior rather than its class. This makes it easier to write generic code that works with a variety of objects.
- Special methods: Python has a number of special methods that allow you to define custom behavior for built-in operations. For example, the
__len__
method allows you to define how thelen()
function works with objects of your class. - Readability: As with the general design principles of Python, classes should also prioritize readability and simplicity. This means using clear and concise names for methods and attributes, avoiding unnecessary complexity, and following PEP 8 guidelines for formatting.
Special methods
Special methods in Python are a set of predefined methods that allow you to define custom behavior for built-in operations on your objects. They are identified by their double underscore prefix and suffix, also known as "dunder" methods.
Here are some of the most commonly used special methods in Python:
__init__(self, ...)
: This is the constructor method that is called when an object is called. It initializes the object's attributes and sets its initial state.__str__(self)
: This methods returns a string representation of the object. It's called by thestr()
function and by theprint()
statement.__repr__(self)
: This method returns a string representation of the object that can be used to recreate the object. It is called by therepr()
function and by the interactive Python shell. The difference between__str__
and__repr__
is that__repr__
is used to create an unambiguous string representation of an object that can be used to recreate the object, while__str__
is used to create a human-readable string representation of an object.__len__(self)
: This method returns the length of the object. It is called by thelen()
function.__getitem__(self, key)
: This method allows you to access an item in the object using bracket notation. It is called when you use the bracket operation([]
) on the object.__setitem__(self, key, value)
: This method allows you to set the value of an item in the object using bracket notation. It is called when you use the bracket operation([]
) on the object with an assignment.__delitem__(self, key)
: This method allows you to delete an item from the object using bracket notation. It is called when you use thedel
statement on the object with bracket notation.__add__(self, other)
: This method allows you to define how the+
operator works with your object. It is called when you use the+
operator on the object.__eq__(self, other)
: This method allows you to define how the==
operator works with your object. It is called when you use the==
operator on the object.__lt__(self, other)
: This method allows you to define how the<
operator works with your object. It is called when you use the<
operator on the object.
There are many other special methods available in Python, but these are some of the most commonly used ones. By defining these methods in your classes, you can customize the behavior of your objects and make them more intuitive and convenient to use.
Example1:
class Person:
def __init__(self, name, age, items):
self.name = name
self.age = age
self.items = items
def __str__(self):
return f"{self.name} ({self.age})"
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
person = Person("Alice", 25)
print(person) # output: Alice (25)
print(repr(person)) # output: Person(name='Alice', age=25)
Example2:
class MyObject:
def __init__(self, items):
self.items = items
def __len__(self):
return len(self.items)
def __getitem__(self, index):
return self.items[index]
def __setitem__(self, index, value):
self.items[index] = value
def __delitem__(self, index):
del self.items[index]
def __add__(self, other):
new_items = self.items + other.items
return MyObject(new_items)
def __eq__(self, other):
if isinstance(other, MyObject):
return self.items == other.items
return False
def __lt__(self, other):
if isinstance(other, MyObject):
return len(self.items) < len(other.items)
return NotImplemented
obj = MyObject([1, 2, 3])
print(len(obj)) # output: 3
print(obj[1]) # output: 2
obj[1] = 4
print(obj.items) # output: [1, 4, 3]
del obj[1]
print(obj.items) # output: [1, 3]
obj1 = MyObject([1, 2])
obj2 = MyObject([3, 4])
obj3 = obj1 + obj2
print(obj3.items) # output: [1, 2, 3, 4]
obj4 = MyObject([1, 2, 3])
obj5 = MyObject([4, 5])
obj6 = MyObject([1, 2, 3])
print(obj1 == obj2) # output: False
print(obj1 == obj3) # output: True
print(obj1 < obj2) # output: False
print(obj2 < obj1) # output: True
built-in functions
- what's len() in len(obj) ?
In the context of len(obj)
, len()
is a built-in Python function that returns the length of an object.
The len()
function can be applied to different types of objects in Python, such as strings, lists, tuples, dictionaries, and sets, and it returns the number of elements in the object.
For example, len("Hello")
returns 5 because there are 5 characters in the string "Hello", and len([1, 2, 3])
returns 3 because there are 3 elements in the list [1, 2, 3]
.
In custom classes, the len()
function can be used to retrieve the length of objects by implementing the __len__()
special method, which should return an integer representing the length of the object.
- more details about built-in Python functions
print()
: This function is used to print the specified message or object to the console. It can take multiple arguments, separated by commas, and can also use formatting to display variables and values.type()
: This function returns the type of an object. It takes one argument and returns the type of that object.len()
: This function returns the length of an object. It can be used with objects such as strings, lists, tuples, dictionaries, and sets.range()
: This function generates a sequence of numbers, starting from a specified start value (by default 0), and incrementing by a specified step value (by default 1), up to but not including a specified stop value.input()
: This function is used to get input from the user via the console. It takes one optional argument, which is the prompt to display to the user, and returns a string containing the user's input.int()
,float()
,str()
: These functions are used to convert values from one type to another.int()
converts a value to an integer,float()
converts a value to a floating-point number, andstr()
converts a value to a string.max()
,min()
: These functions are used to find the maximum or minimum value in a sequence. They can be used with objects such as lists, tuples, and sets.sum()
: This function is used to find the sum of all the values in a sequence. It can be used with objects such as lists, tuples, and sets.sorted()
: This function is used to sort a sequence in ascending order. It can be used with objects such as lists, tuples, and sets.zip()
: This function is used to combine two or more sequences into a single sequence of tuples. It takes two or more arguments and returns a zip object containing tuples of elements from the input sequences.
These are just a few examples of the built-in Python functions. Python has many more built-in functions that can be used to perform a variety of tasks, such as manipulating strings, performing mathematical calculations, working with files and directories, and more.
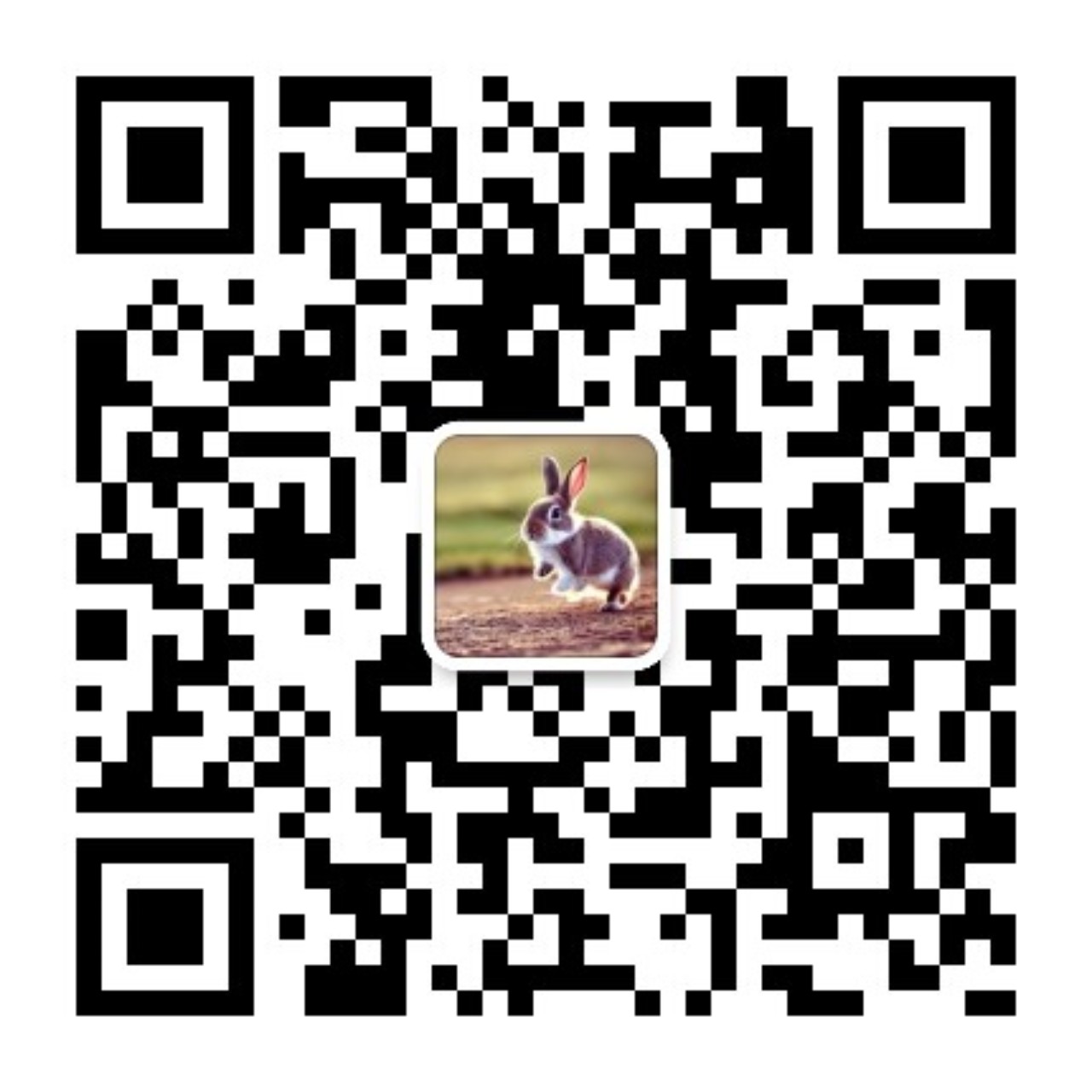
關註我的公眾號 不定期推送資訊,接受私信許願
作者:iSherryZhang 出處:https://www.cnblogs.com/shuezhang/ 本文版權歸作者和博客園共有,歡迎轉載,但未經作者同意必須保留此段聲明,且在文章頁面明顯位置給出原文連接,否則保留追究法律責任的權利。