家居網購項目實現08 以下皆為部分代碼,詳見 https://github.com/liyuelian/furniture_mall.git 19.功能18-添加家居到購物車 19.1需求分析/圖解 會員登錄後,可以添加家居到購物車 完成購物車的設計和實現 每添加一個家居,購物車的數量+1並顯示 1 ...
家居網購項目實現08
以下皆為部分代碼,詳見 https://github.com/liyuelian/furniture_mall.git
19.功能18-添加家居到購物車
19.1需求分析/圖解
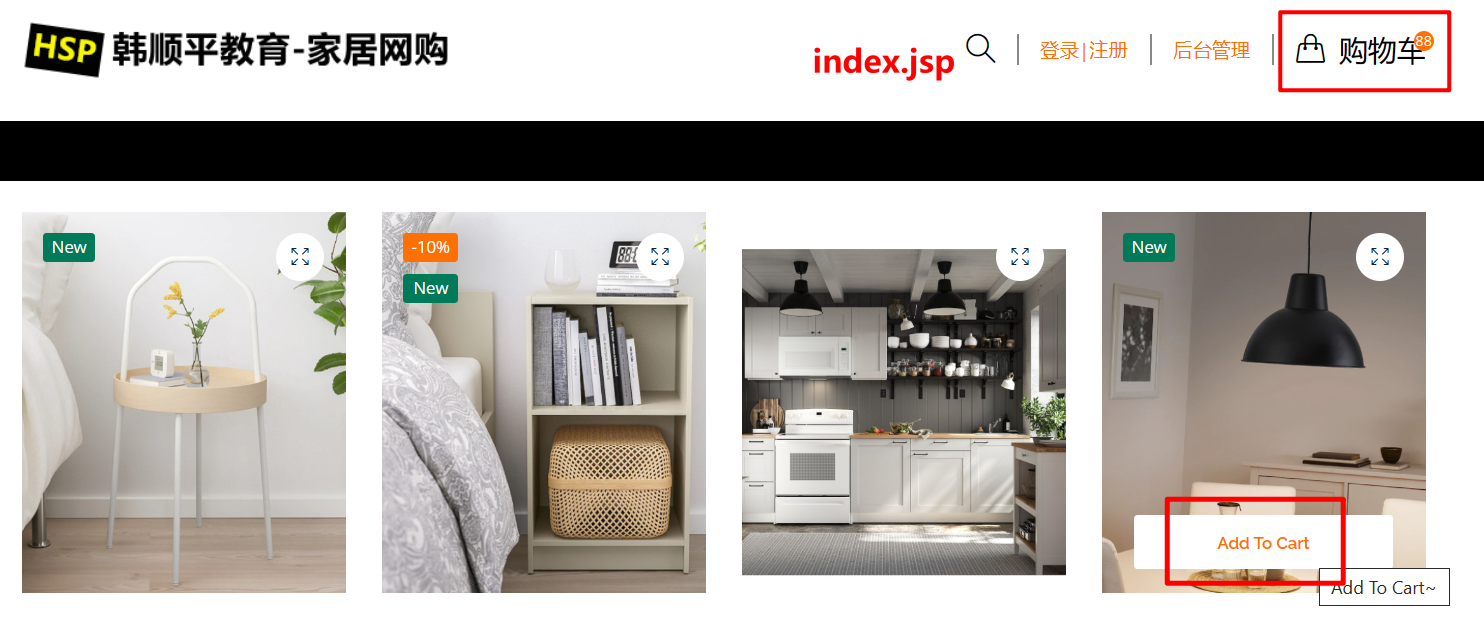
- 會員登錄後,可以添加家居到購物車
- 完成購物車的設計和實現
- 每添加一個家居,購物車的數量+1並顯示
19.2思路分析
說明:這裡實現的購物車是session版的,不是資料庫版的。也就是說,用戶購物車的數據在退出登錄或者退出瀏覽器後將會清空。
如果希望將購物車放到mysql中,將Cart數據模型改成一張表即可,即Entity和表的一種映射概念,你可以使用Entity-DAO-Service。大概做法就是購物車表和CartItem實體映射,表的一行記錄就是一個cartItem類。通過記錄用戶id和用戶聯繫起來。
.png)
JavaEE+mvc模式:
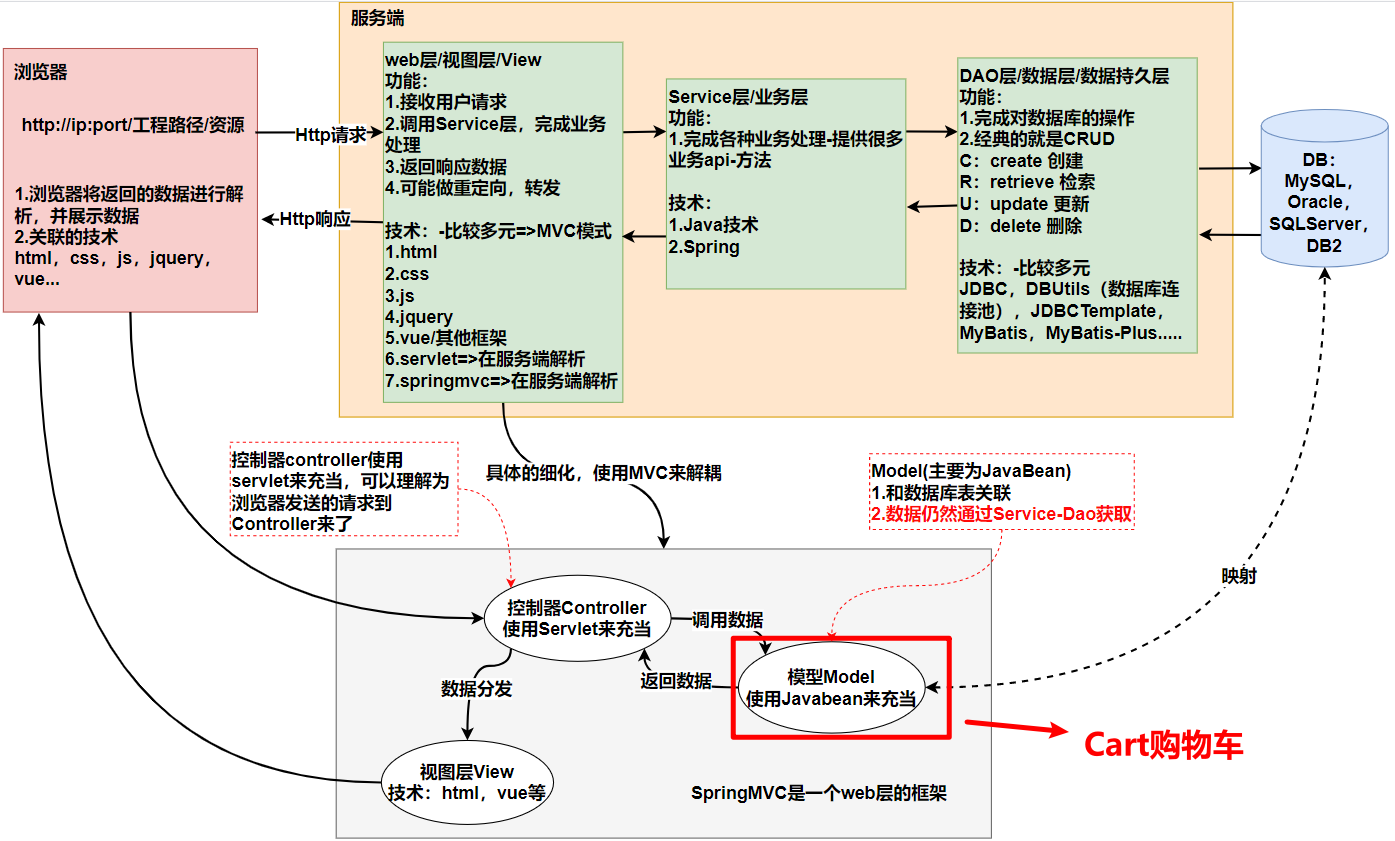
19.3代碼實現
19.3.1entity層
CartItem.java
package com.li.furns.entity;
import java.math.BigDecimal;
/**
* CartItem 表示購物車的一項,就是某個家居數據
*
* @author 李
* @version 1.0
*/
public class CartItem {
//定義屬性->根據需求
private Integer id; //家居id
private String name; //家居名
private BigDecimal price; //家居單價
private Integer count; //家居數量
private BigDecimal totalPrice; //總價格
public CartItem() {
}
public CartItem(Integer id, String name, BigDecimal price, Integer count, BigDecimal totalPrice) {
this.id = id;
this.name = name;
this.price = price;
this.count = count;
this.totalPrice = totalPrice;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
public Integer getCount() {
return count;
}
public void setCount(Integer count) {
this.count = count;
}
public BigDecimal getTotalPrice() {
return totalPrice;
}
public void setTotalPrice(BigDecimal totalPrice) {
this.totalPrice = totalPrice;
}
@Override
public String toString() {
return "CartItem{" +
"id=" + id +
", name='" + name + '\'' +
", price=" + price +
", count=" + count +
", totalPrice=" + totalPrice +
'}';
}
}
Cart.java
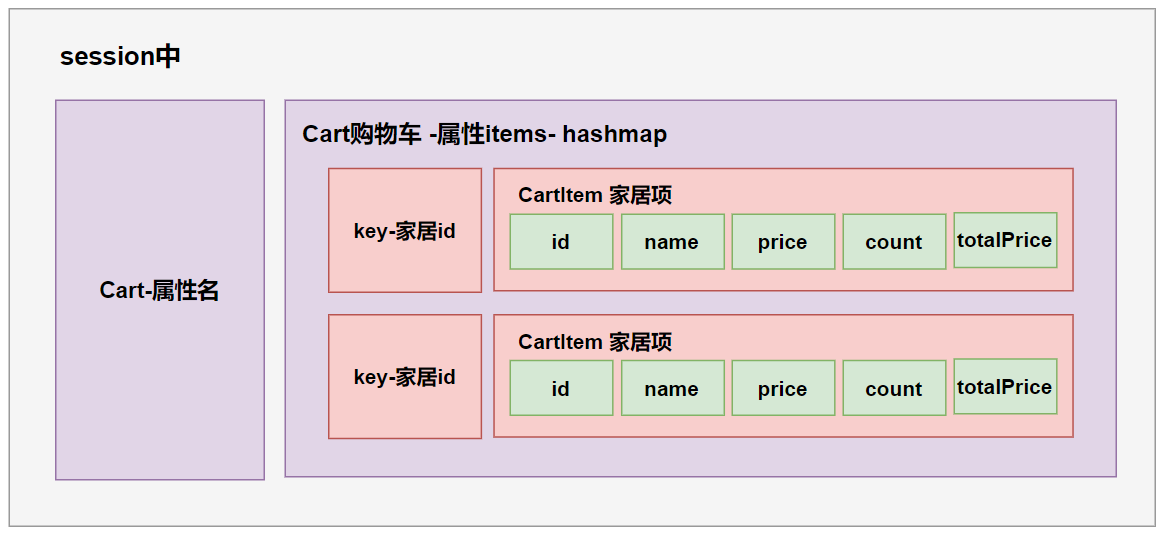
package com.li.furns.entity;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.Set;
/**
* Cart就是一個購物車,包含很多CartItem對象
*
* @author 李
* @version 1.0
*/
public class Cart {
//定義屬性
//包含多個CartItem對象,使用HashMap來保存
private HashMap<Integer, CartItem> items = new HashMap<>();
//添加家居CartItem到Cart
public void addItem(CartItem cartItem) {
//添加cartItem到Cart前要先判斷-該item是第一次添加還是二次以後添加
//使用家居id在items中找有沒有對應家居
CartItem item = items.get(cartItem.getId());
if (null == item) {//說明當前購物車還沒有這個cartItem
//添加該cartItem到購物車Cart中去
items.put(cartItem.getId(), cartItem);
} else {//當前購物車已經有這個cartItem
//數量增加1
item.setCount(item.getCount() + 1);
//修改總價
//item.setTotalPrice(item.getPrice().multiply(new BigDecimal(item.getCount())));
item.setTotalPrice(item.getTotalPrice().add(item.getPrice()));
}
}
@Override
public String toString() {
return "Cart{" +
"items=" + items +
'}';
}
}
19.3.2test
CartTest.java
package com.li.furns.test;
import com.li.furns.entity.Cart;
import com.li.furns.entity.CartItem;
import org.junit.jupiter.api.Test;
import java.math.BigDecimal;
/**
* @author 李
* @version 1.0
*/
public class CartTest {
private Cart cart = new Cart();
@Test
public void addItem() {
cart.addItem(new CartItem(1, "沙發", new BigDecimal(10), 2, new BigDecimal(20)));
cart.addItem(new CartItem(2, "小椅子", new BigDecimal(20), 2, new BigDecimal(40)));
System.out.println("cart=>" + cart);
}
}
19.3.3web層
配置CartServlet
<servlet>
<servlet-name>CartServlet</servlet-name>
<servlet-class>com.li.furns.web.CartServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>CartServlet</servlet-name>
<url-pattern>/cartServlet</url-pattern>
</servlet-mapping>
CartServlet.java
package com.li.furns.web;
import com.li.furns.entity.Cart;
import com.li.furns.entity.CartItem;
import com.li.furns.entity.Furn;
import com.li.furns.service.FurnService;
import com.li.furns.service.impl.FurnServiceImpl;
import com.li.furns.utils.DataUtils;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @author 李
* @version 1.0
*/
public class CartServlet extends BasicServlet {
//增加一個屬性
private FurnService furnService = new FurnServiceImpl();
/**
* 添加家居數據到購物車
*
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void addItem(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//得到添加的家居ID
int id = DataUtils.parseInt(req.getParameter("id"), 0);
//獲取到id對應的Furn對象
Furn furn = furnService.queryFurnById(id);
if (furn == null) {//說明沒有查到對應的家居信息
return;
//todo
}
//根據furn構建CartItem
CartItem item =
new CartItem(furn.getId(), furn.getName(), furn.getPrice(), 1, furn.getPrice());
//從session獲取cart對象
Cart cart = (Cart) req.getSession().getAttribute("cart");
if (null == cart) {//說明當前的session沒有cart對象
//創建一個cart對象
cart = new Cart();
//將其放入到session中
req.getSession().setAttribute("cart", cart);
}
//將cartItem加入到cart對象
cart.addItem(item);
//添加完畢後需要返回到添加家居的頁面
String referer = req.getHeader("Referer");
resp.sendRedirect(referer);
}
}
19.3.4修改前端介面
views/cutomer/index.jsp
點擊add to cart按鈕,添加家居信息到購物車中
<script type="text/javascript">
$(function () {
//給add to cart綁定事件
$("button.add-to-cart").click(function () {
//獲取到點擊的furn-id
var furnId = $(this).attr("furnId");
//發出一個請求-添加家居=>後面改成ajax
location.href = "cartServlet?action=addItem&id=" + furnId;
})
})
</script>
顯示購物車信息

19.4完成測試
未添加家居前購物車顯示
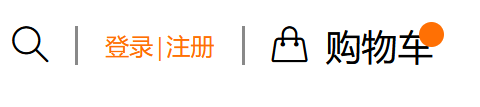
點擊add to cart,添加家居到購物車
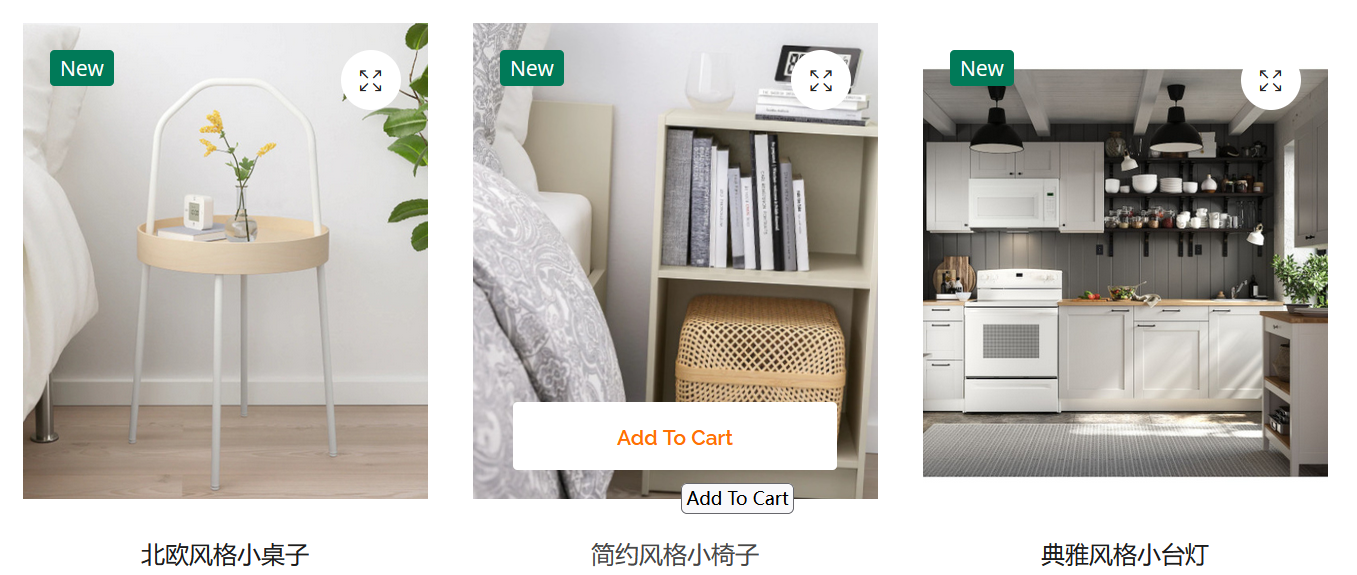

添加多個家居信息
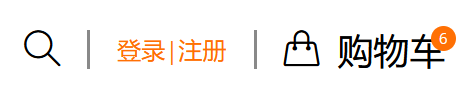
20.功能19-顯示購物車
20.1需求分析/圖解
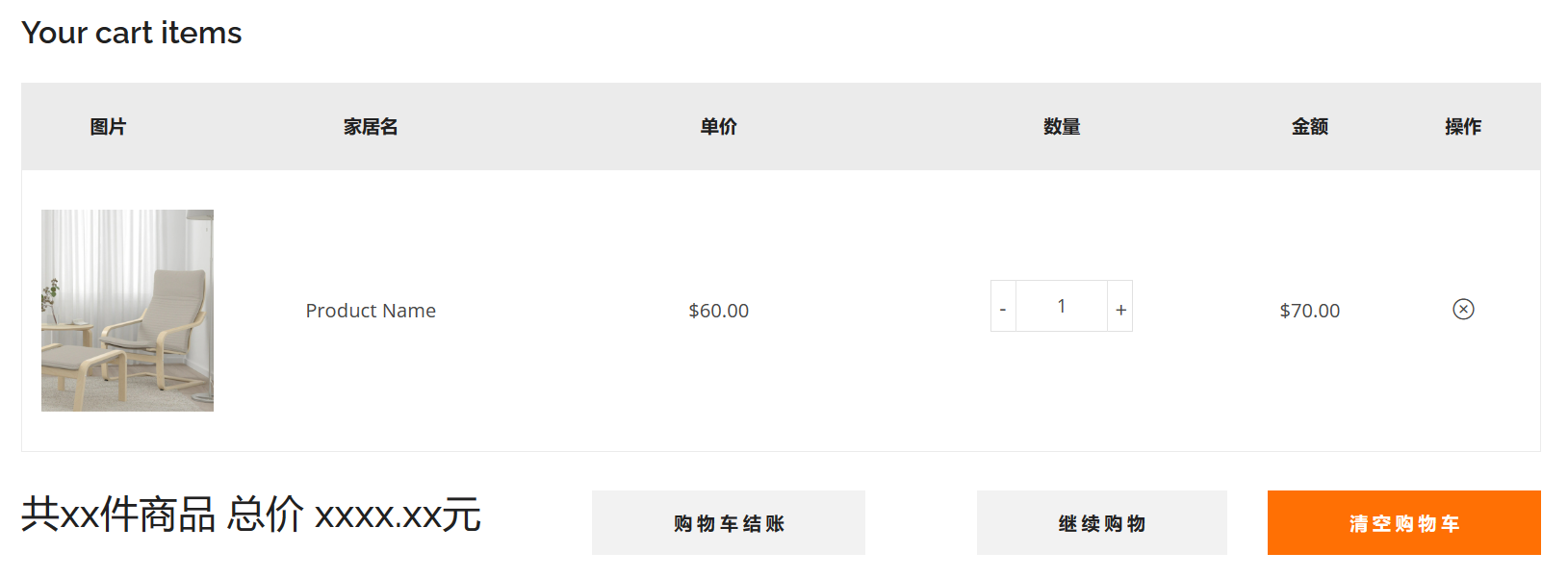
- 查看購物車,可以顯示如上信息
- 選中了哪些家居,名稱,數量,金額
- 統計購物車共多少商品,總價多少
20.2思路分析
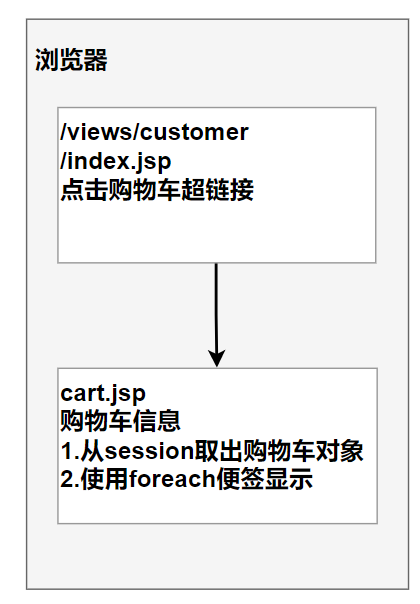
20.3代碼實現
Cart.java
為了配合前端介面,增加一些方法
public HashMap<Integer, CartItem> getItems() {
return items;
}
public BigDecimal getCartTotalPrice() {
BigDecimal cartTotalPrice = new BigDecimal(0);
//遍歷購物車,返回整個購物車的商品總價格
Set<Integer> keys = items.keySet();
for (Integer id : keys) {
CartItem item = items.get(id);
//一定要把add後的值重新賦給cartTotalPrice
cartTotalPrice = cartTotalPrice.add(item.getTotalPrice());
}
return cartTotalPrice;
}
public int getTotalCount() {
//因為前端每點擊一次添加商品,購物車顯示就會調用getTotalCount方法,
//如果不置0,數量相當是重覆添加
int totalCount = 0;
//遍歷購物車,返回商品總數量
Set<Integer> keys = items.keySet();
for (Integer id : keys) {
totalCount += ((CartItem) items.get(id)).getCount();
}
return totalCount;
}
用foreach在cart.jsp迴圈顯示購物車信息
<!-- Cart Area Start -->
<div class="cart-main-area pt-100px pb-100px">
<div class="container">
<h3 class="cart-page-title">Your cart items</h3>
<div class="row">
<div class="col-lg-12 col-md-12 col-sm-12 col-12">
<form action="#">
<div class="table-content table-responsive cart-table-content">
<table>
<thead>
<tr>
<th>圖片</th>
<th>家居名</th>
<th>單價</th>
<th>數量</th>
<th>金額</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<%--找到顯示的購物車項,進行迴圈顯示--%>
<c:if test="${not empty sessionScope.cart.items}">
<%--
1.items實際上是HashMap<Integer, CartItem>
2.所以通過foreach標簽取出的每一個對象entry 是 HashMap<Integer, CartItem> 的k-v
3.因此var其實就是entry
4.所以要取出cartItem是通過entry.value
--%>
<c:forEach items="${sessionScope.cart.items}" var="entry">
<tr>
<td class="product-thumbnail">
<a href="#"><img class="img-responsive ml-3"
src="assets/images/product-image/1.jpg"
alt=""/></a>
</td>
<td class="product-name"><a href="#">${entry.value.name}</a></td>
<td class="product-price-cart"><span class="amount">${entry.value.price}</span></td>
<td class="product-quantity">
<div class="cart-plus-minus">
<input class="cart-plus-minus-box" type="text" name="qtybutton"
value="${entry.value.count}"/>
</div>
</td>
<td class="product-subtotal">${entry.value.totalPrice}</td>
<td class="product-remove">
<a href="#"><i class="icon-close"></i></a>
</td>
</tr>
</c:forEach>
</c:if>
</tbody>
</table>
</div>
<div class="row">
<div class="col-lg-12">
<div class="cart-shiping-update-wrapper">
<h4>共${sessionScope.cart.totalCount}件商品 總價 ${sessionScope.cart.cartTotalPrice}元</h4>
<div class="cart-shiping-update">
<a href="#">購 物 車 結 賬</a>
</div>
<div class="cart-clear">
<button>繼 續 購 物</button>
<a href="#">清 空 購 物 車</a>
</div>
</div>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
<!-- Cart Area End -->
20.4完成測試
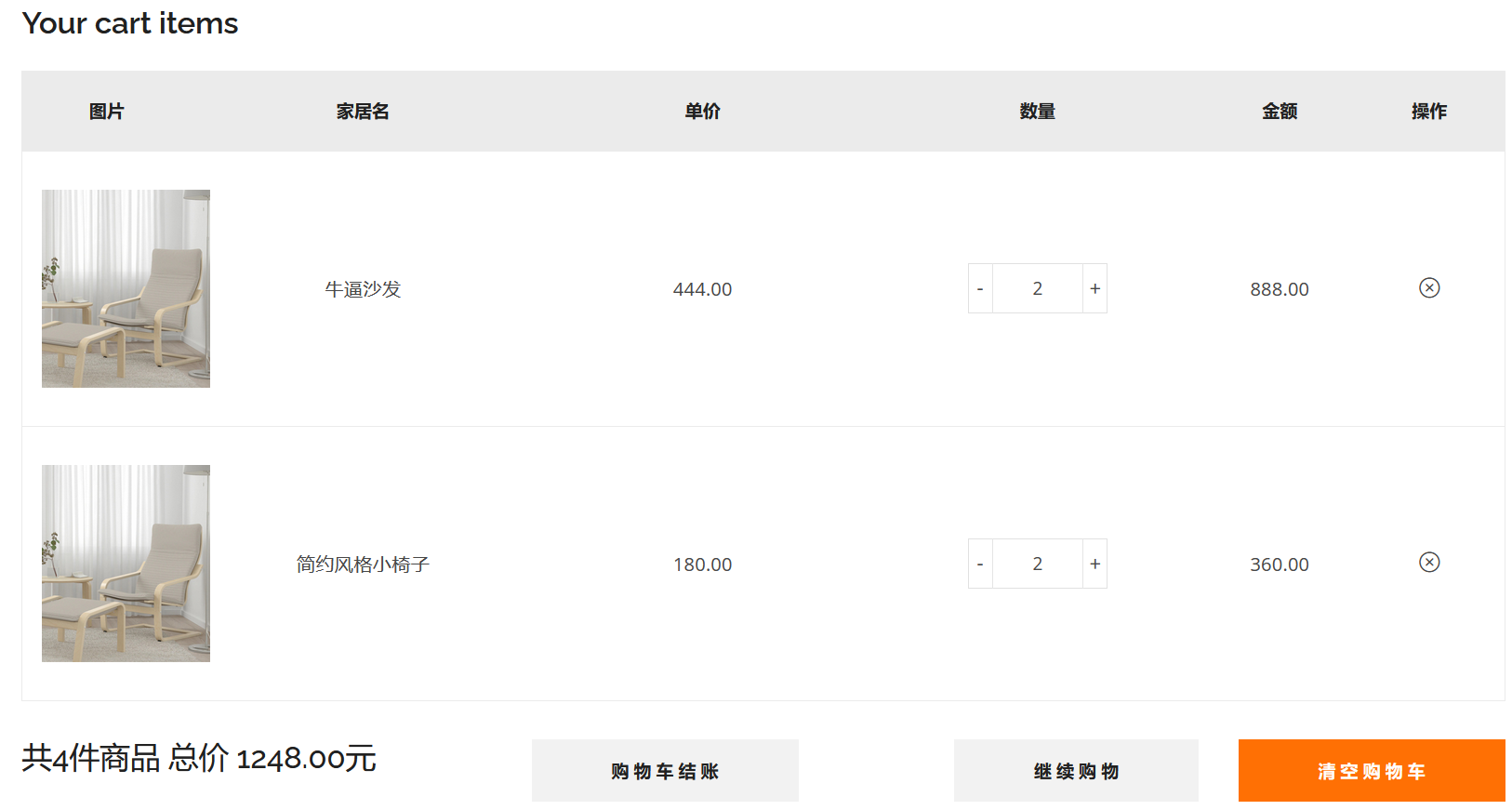
21.功能20-修改購物車
21.1需求分析/圖解
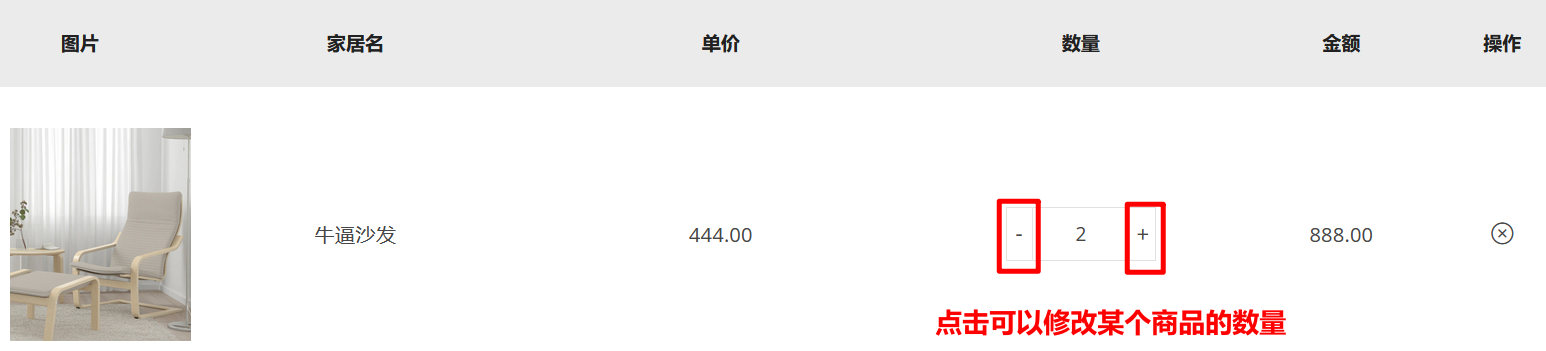
- 進入購物車頁面,可以修改購買數量
- 更新該商品的金額
- 更新購物車商品數量和總金額
21.2思路分析
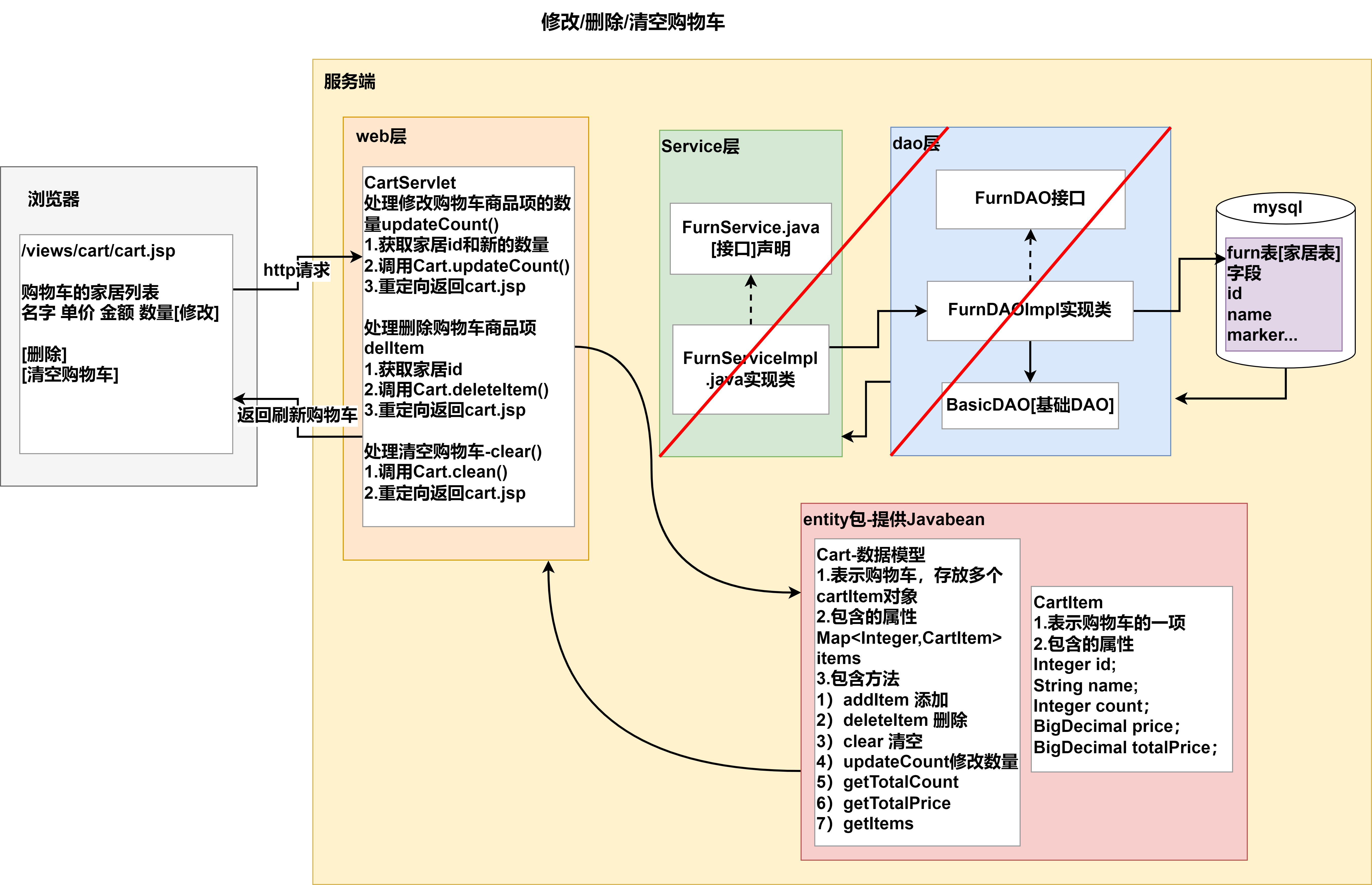